I want to use the deploymented c++ library on windows.
The sample example-standalone-inferencing code only tell me to copy raw features from live classification. But do not have how to generate raw features.
For (Generate Raw Features from Audio using API) questions, you said we can use python_speech_features, I tried:
from python_speech_features import mfcc
import scipy.io.wavfile as wav
(sr, y) = wav.read(“a.wav”)
feat = mfcc(y, sr)
feat = feat.flatten()
but this is not the raw features, right? This value can’t be used as the input value for run_classifier function.
How can I just generate the Raw Features of a wav file? Can you give me some sample code? Thanks.
Hi @mzymzy2009,
The easiest way to get raw features for testing the example-standalone-inferencing code is to go to your project, go to the Model testing page, click the 3 dots next to a sample, and click Show classification. From there, click the Copy button next to Raw features.
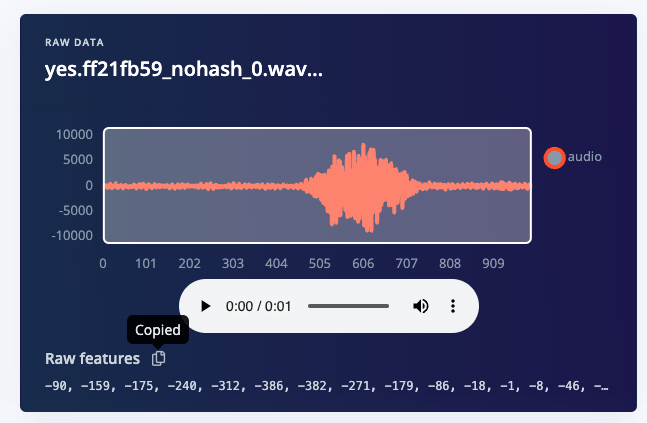
Paste that list of numbers into the features[]
array in your example-standalone-inferencing code.
I know that, I already tested. I just want to use it on my project.
I just want to know how to generate the Raw Features of a wav file!
Can you give me some sample code, thanks.
Hi @mzymzy2009,
You do not need to recompute the preprocessing/DSP features when you deploy your impulse. Your “processing block” is already included in the run_classifier()
function call. For example, if you are using MFCCs, you do not need to calculate MFCCs. You just need to pass in raw audio readings (int16), which is what we call “raw features.”
If you are using Python and you want to generate these raw features, you just need to read your .wav file, truncate the array to your desired input (e.g. 16000 samples if you use a 1 sec window at 16 kHz sample rate), and then print out the raw features. For example:
from scipy.io.wavfile import read
import numpy as np
# Load .wav file into NumPy array
wav = np.array(read("yes-01.wav")[1], dtype=int)
# Truncate as needed (e.g. for 1 sec window at 16 kHz sample rate)
wav = wav[:16000]
# Print "raw features"
for i in range(len(wav)):
if i < len(wav) - 1:
print(f"{wav[i]}, ", end="")
else:
print(f"{wav[i]}")